Welcome to the Fall CTF 2024 Guide! Feel free to click around the categories as you solve challenges.
Hello Fall CTF Developers!!!!
When developing exploits for various challenges, it might be beneficial to use scripts and automation to make your life easier. Especially when you want to deal with sending bytes and data that are not printable, or large numbers when dealing with cryptography.
Of course, one of the easiest scripting languages to use is Python. While you could manually set up connections, text piping, and the whole host of annoying debugging issues that come along with that, there's already a handy library to handle the plumbing for you.
pwntools
is a Python library with lots of handy functions, classes, and scripts to help automate and streamline exploit development.
You can install pwntools through pip
, Python's package manager:
pip3 install pwntools
or
python3 -m pip install pwntools
If the above command does not work for you on MacOS or Linux, you may need some dependencies that are required to install pwntools. Specifically, pwntools expects you to have cmake
and pkg-config
. Try running:
brew install cmake
brew install pkg-config
or
sudo apt-get install cmake
sudo apt-get install pkg-config
pwntools
uses the idea of "tubes" to handle data transfer/receive. You can make a connection with an actual network interface (like you would with netcat
), or with a local process, and link standard in and standard out with pwntools
.
Here's a few ways to make a connection:
from pwn import * # It's usually just better to import everything when using pwntools
# Start a process on your machine, locally
conn = process('./file')
# Start a process and simultaneously start gdb debugging it.
# You can provide an initial set of commands using the gdbscript parameter
conn2 = gdb.debug('./file', gdbscript="""
b main
continue
""")
# Pause a process running locally and attach gdb to it and start debugging
gdb.attach(conn)
# Connect to a server
port = 22
conn3 = remote('ip.or.domain', port)
With process
, it just launches the file specified via the path. If you give it an array of strings, you can run a file with command line arguments, e.g. ['./file', '-t', 'data.txt']
. You can also start a program locally with a debugger using gdb.debug()
instead. You need to be using a terminal with support for paneling. So install and enter terminal multiplexer such as tmux
before running a debugger with pwntools (Windows Terminal, oddly, supports this natively?). You can also attach gdb to an existing process with gdb.attach()
. Finally, for communicating with networks (and in our case, challenge servers), you can use remote()
to provide an ip/domain and port to connect to.
Once we have our connection, we can send or receive data from it. There are many functions to do this, but I'll show off a few:
from pwn import *
port = 9999
conn = remote('chal.sigpwny.com',port)
# Receive functions will return a bytestring of what it receives.
# Receive text until it encounters a new line character (e.g. \n)
line = conn.recvline()
# Receive text until it encounters the specified delimiter.
prompt = conn.recvuntil(b'>> ')
# Receive exactly n bytes.
resp = conn.recvn(8)
# Receive everything until End of File (EOF) is received from the pipe (i.e. they closed the connection)
everything = conn.recvall()
# Sends the string along with an new line ending
conn.sendline(b'my response\x05\x0f\xa0')
# Does NOT send a newline
conn.send(b'not a line yet')
# Does a recvuntil('delim') and sends the 2nd argument
conn.sendlineafter(b'>> ', b'1')
Note that strings in these functions are bytestrings b''
. pwntools will warn you if you dont use bytestrings, and automatically convert to ASCII.
That being said, this means its really easy to send non-printable characters over the wire using \x
escape. It's also important to keep in mind that functions looking for a specific character (like a new line or delimiter), will block until it does. If your script is stuck, check to see whether or not you are actually getting the text you think you are. Running the script with the DEBUG argument will cause it to print a hex dump of any data it sends/receives: python3 exploit.py DEBUG
Note, pipes with processes and such are buffered. The OS maintains a 4kb buffer of any text received. If you call recvline()
, and the program sent 3 lines in total, only the first line is retrieved, and the other 2 remain in the buffer. Keep this in mind when processing received data. If you want to retrieve anything that's in the buffer currently, recv()
will empty it out.
Finally, if you want to regain control of the process input/output, simply call:
conn.interactive()
You'll want this to read the final printout of a program after running your exploit, or to use a shell, etc.
For some of our challenges, you will need to use pwntool
s asm
function. This requires a binutils installation for the architecture that our machines are running on, in this case, amd64. If you are on an M1/M2 mac, you'll need to run the following commands somewhere to generate assembly for our servers:
wget https://raw.githubusercontent.com/Gallopsled/pwntools-binutils/master/macos/binutils-amd64.rb
brew install binutils-amd64.rb
The miscellaneous category contains challenges not found in the other usual categories. Common types of challenges are jails and various programming/algorithm problems. Jails are programs that give the user some degree of control, with the goal to break out of the particular restrictions of the jail.
One common challenge format is the Python jail, or "pyjail" for short. These are Python programs that take input form the user and executes it directly as Python code in some form. Often times there will be restrictions on the Python code that is executed. See here for some ideas.
As a general tip, running the file
command on challenge files can tell you a lot of useful information. One file format in many misc challenges is the .wav format, which stores audio data with pulse-code modulation. In this format, audio waveforms are sampled at discrete intervals and are stored as a series of integers.
We have run two web meeting this semester:
- Web 1, covering HTML, CSS, and Javascript
- Web 2, covering SQLi and XSS
Websites use three main languages: HTML, CSS, and Javascript. HTML is the skeleton of the website, and organizes each of the different elements onto the user's screen. CSS is how you edit and develop the styles on a website. The most important and widely used language within the web is Javascript. Javascript allows you to dynamically change elements within your site, have something happen when a button is pressed, or make a requests to other computers.
When you click on a link within your browser, your computer makes a request to a server located at the address of the link you clicked. This request is then processed on the server's side, and the server sends back the webpage you want to load. This is the Client-Server Model. By manipulating processes within this model's process, you can access extra content on either the server or client side!
When content is sent between your computer and the server, it includes additional metadata called "Headers". Some of this data remains in your browser, either as cookies or local storage (technically more kinds).
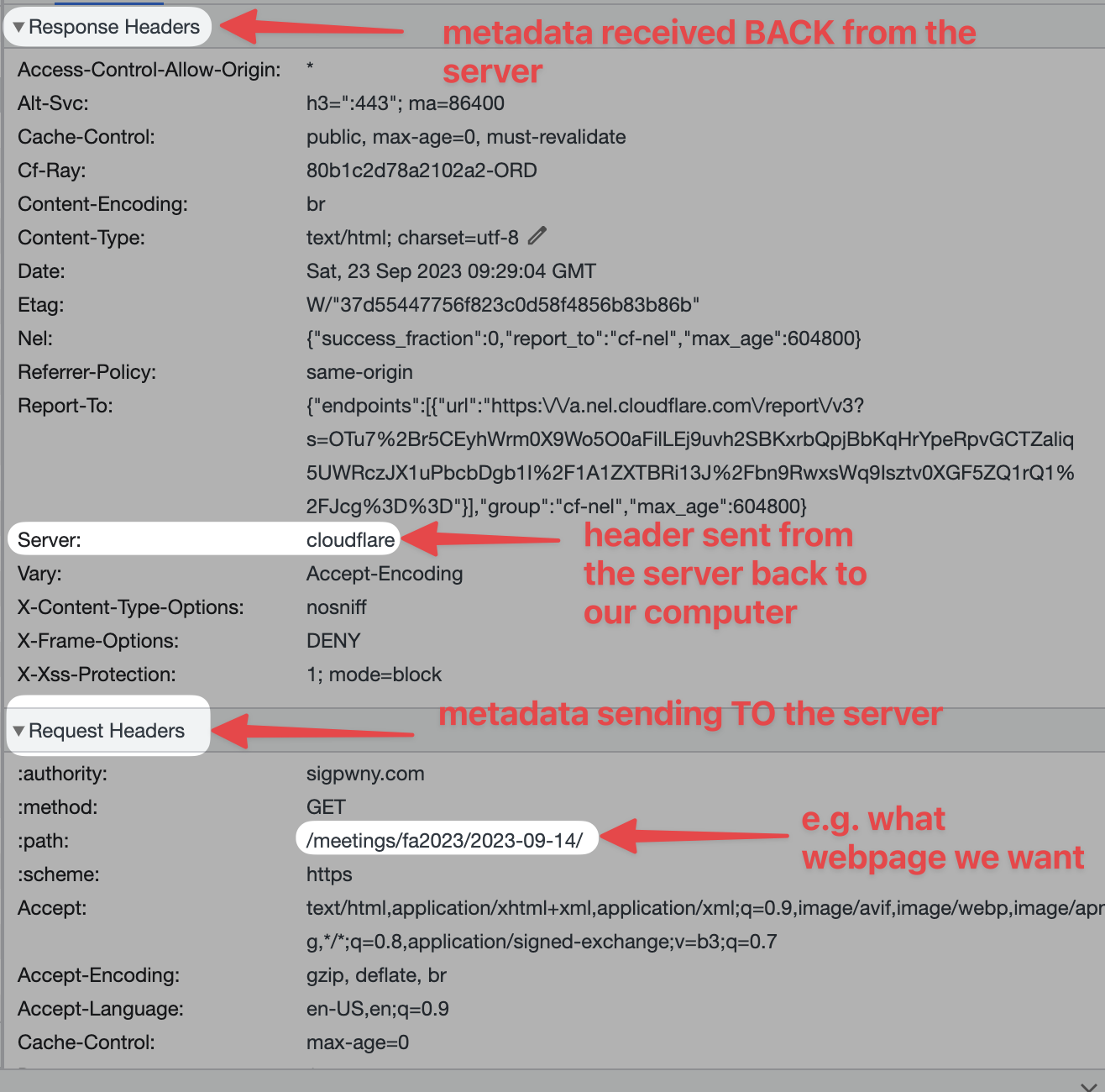
- Cookies are saved per website, and are sent in each request. They can be changed by Javascript or a request header.
- Local Storage is saved per website, but are not sent in each request. They can be changed by Javascript in your browser.
Developer tools is how you view additional website about an information. For our challenges, we reccommend you download Chrome or Firefox, and not use Safari.
To open devtools, hit Ctrl + Shift + C
(windows) or Command + Shift + C
(mac). Alternatively, right click and hit inspect.
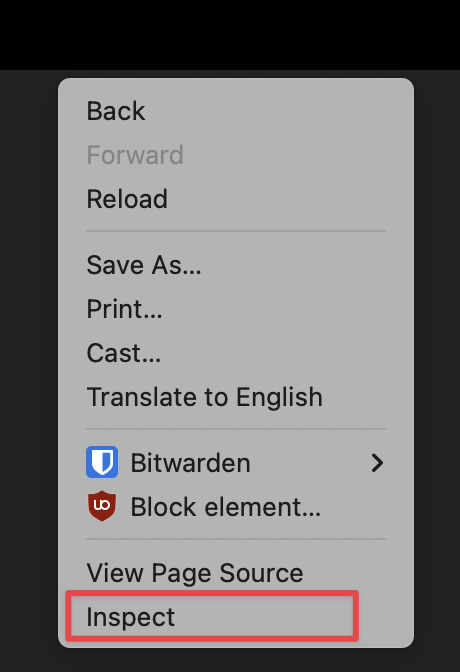
Chrome Devtools is a suite of software developer information for web development. During challenges, you will be able to poke around different tabs. Here are some helpful tabs to lookout for:
- Console (you can run your own javascript in this tab)
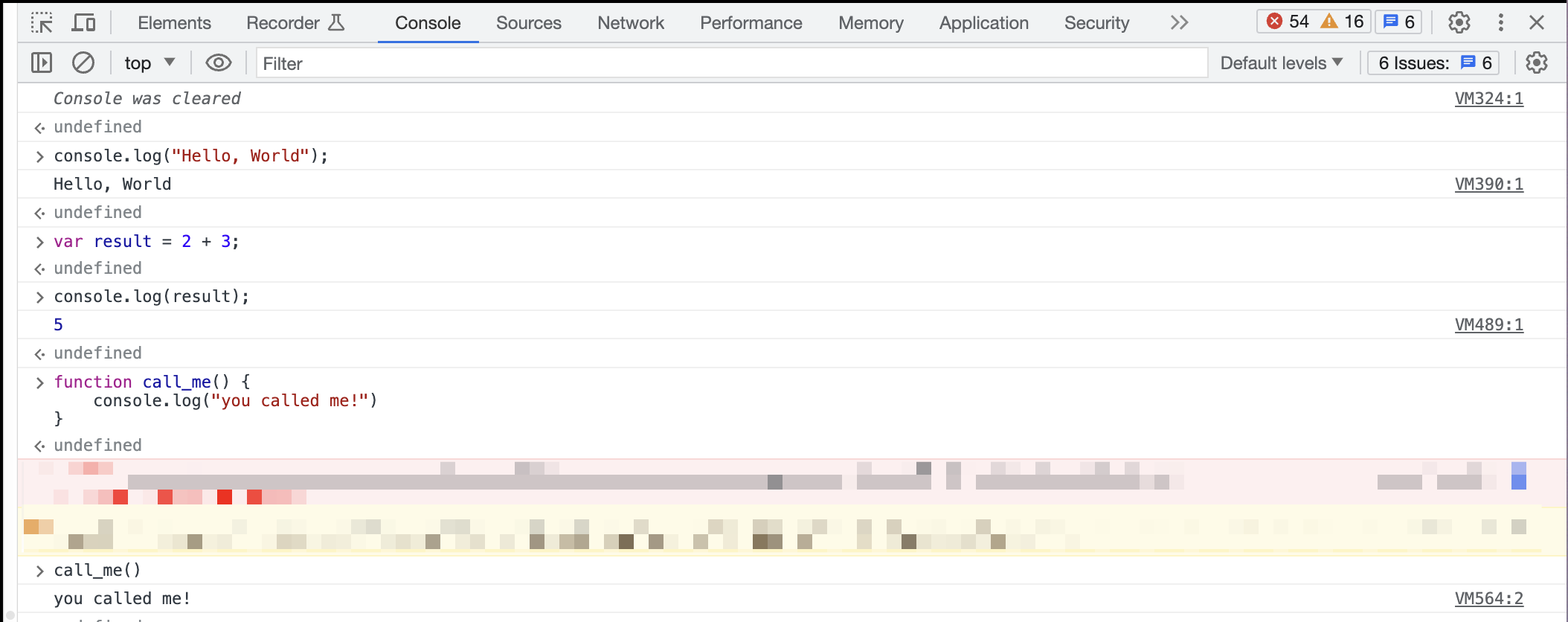
Pro Tip: You can use breakpoints within the console by clicking next to the line number. This can allow you to stop at certain lines before the run and check variables
The network tab shows all information transmitted to/from your computer to the server (website).

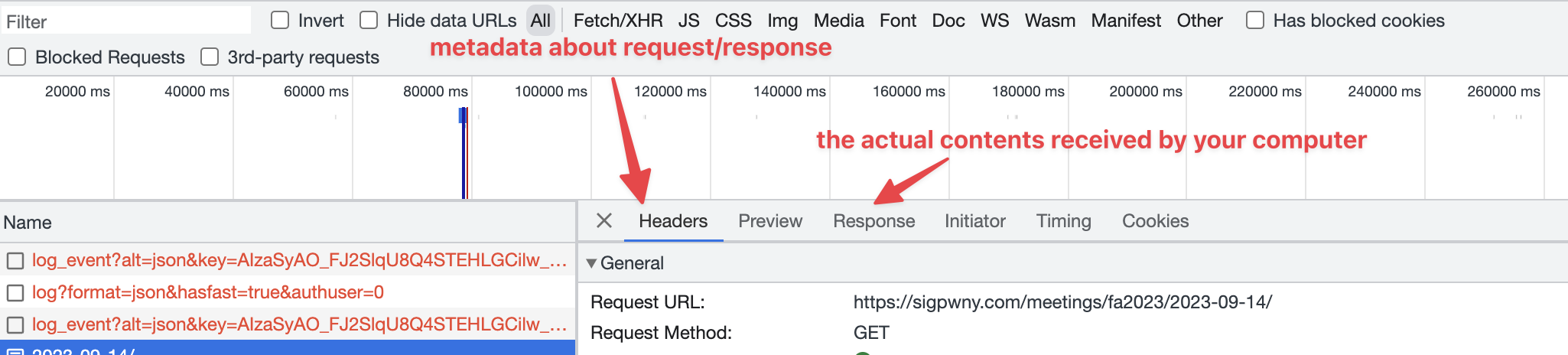
The sources tab shows a listing of all files on the server that were requested.
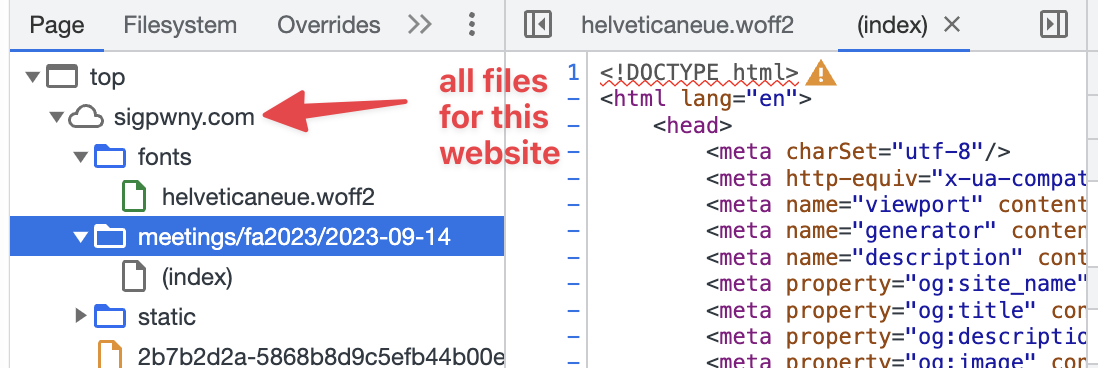
The application tab shows the saved cookies, local storage, and other information stored in your browser.
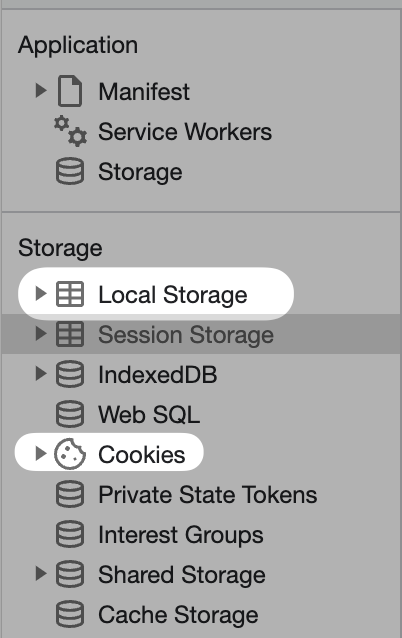
This is not an exhaustive list, but just a few useful tabs within Devtools.
base64 - Looks like this
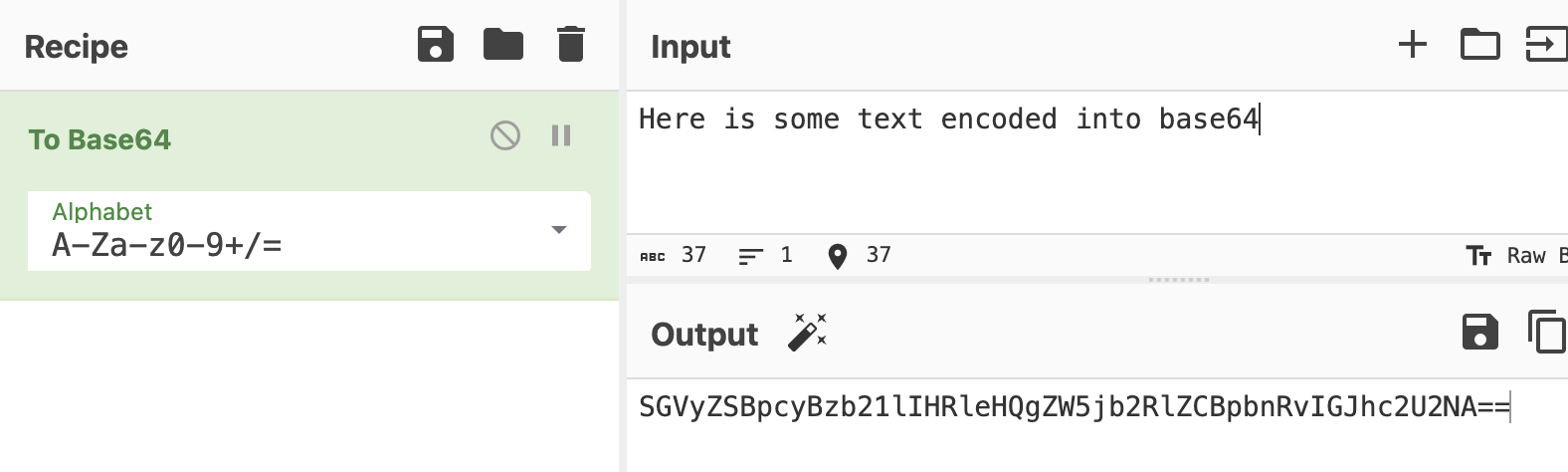
url encoding - Looks like this
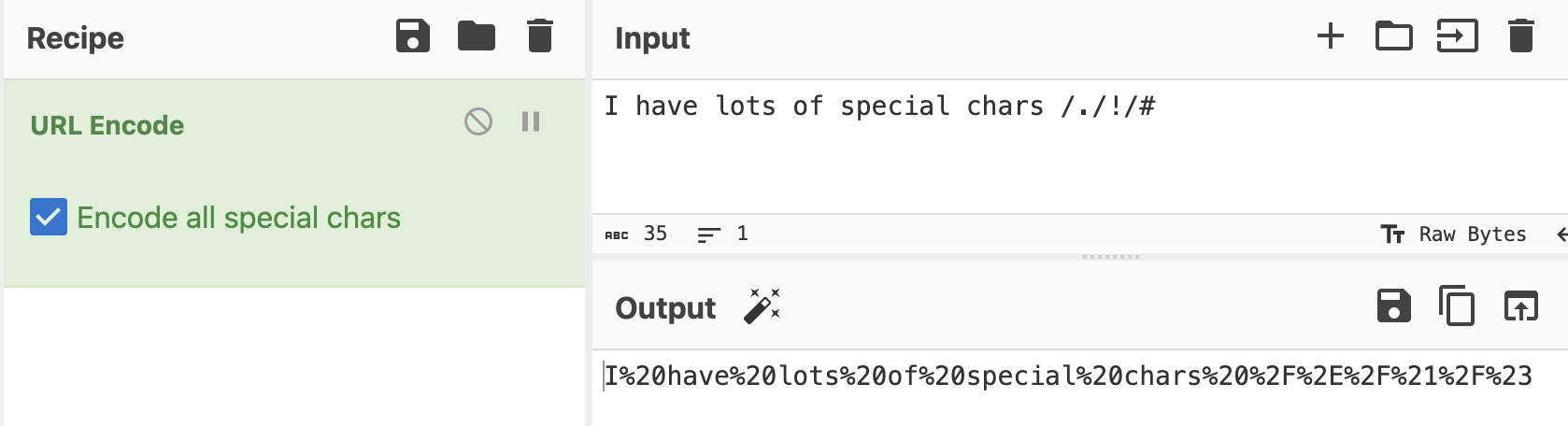
You can use CyberChef to decode.
More in-depth explanations can be found in the Web 2 slides about SQL.
SQL, or Structured Query Language is a language for fetching information from a server.
For example,
SELECT netid, firstname FROM students WHERE lastname = "Tables"
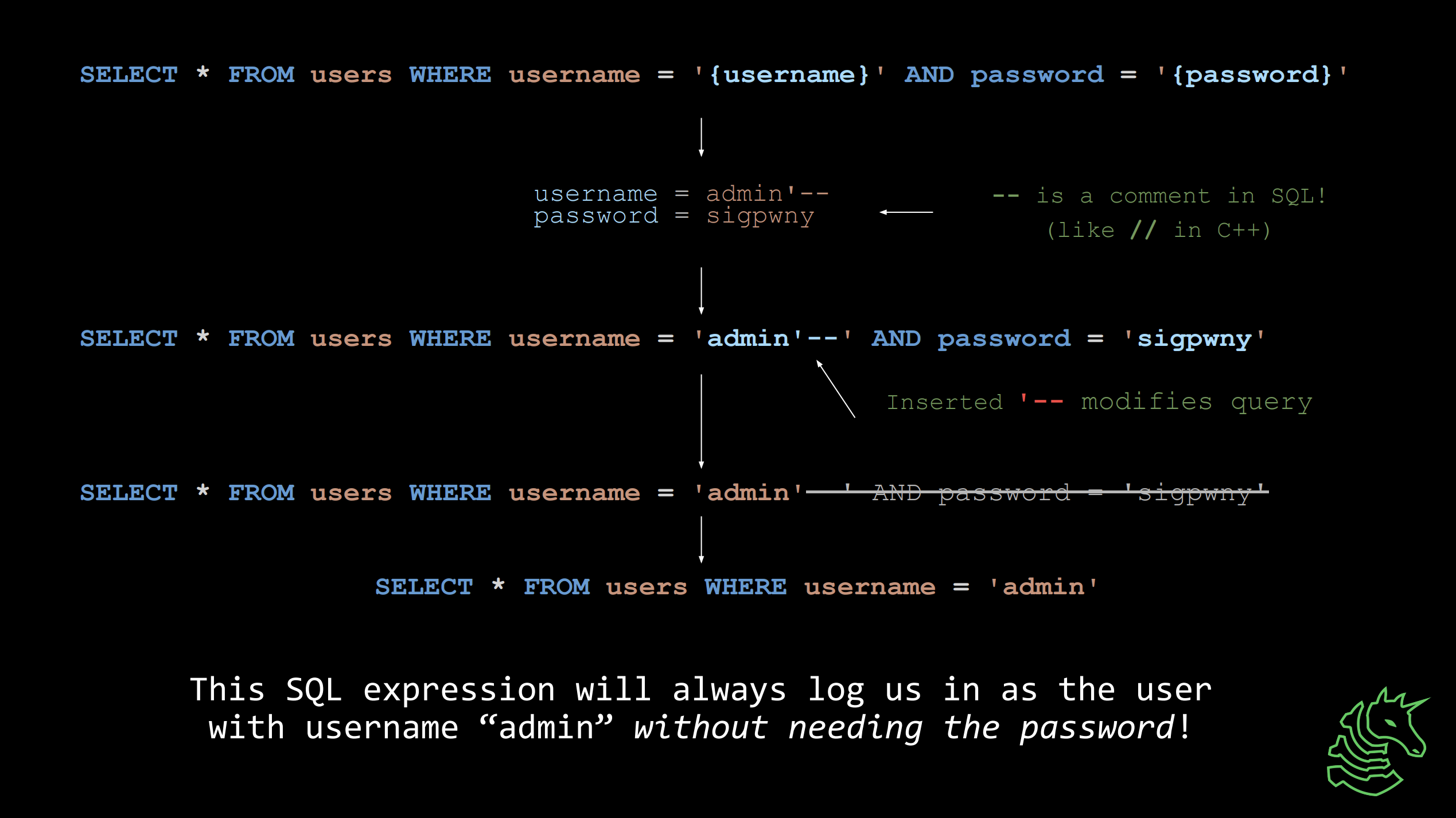
If code is written incorrectly, you can modify an SQL Statement as shown above.
More details on SQL: https://portswigger.net/web-security/sql-injection
Resource on SQL Union Attack: https://portswigger.net/web-security/sql-injection/union-attacks
Command Injection lets you execute multiple linux commands at the same time. It is very similar to SQL Injection, except instead of
changing a database query, you are changing commands executed in the command line.
For example, in your terminal, you are able to execute multiple commands using the ;
ability
$> echo "command 1"; echo "command 2"
command 1
command 2
If you are able to "inject" something directly into the command you are executing, you can make it do additional things.
$> echo "YOUR INPUT"
If I had set YOUR INPUT
to -HI"; ls ; "BYE-
then the command would look like
echo "-HI"; ls; "BYE-"
Some useful commands are:
ls
- list files
cat x.txt
- output the contents of the file x.txt
If you want more resources on learning the linux command line...
Cross-Site Scripting is a vulnerability that allows an attacker to execute malicious scripts on a website. This can be used to steal cookies, redirect users to malicious websites, or deface the website.
I like to think of XSS as as another type of injection attack, but instead of injecting into a database or command line, you're injecting into the website's HTML.
For example, let's say you have a website that displays a user's name on the page. If the website includes the input directly into the HTML, you can put your own HTML code in the input and have it execute on the page.
<p>Welcome, <span id="username">USER INPUT</span></p>
If I had set USER INPUT
to <script>alert("Hello!")</script>
, then the website would display a popup saying "Hello!".
<p>Welcome, <span id="username"><script>alert("Hello!")</script></span></p>
More details on XSS: https://portswigger.net/web-security/cross-site-scripting
A useful resource for receiving requests is webhook.site. For example, if you need to extract some data from a website, you can have your XSS payload send a request to your webhook.site URL with the data you need.
Be careful when exfiltrating data to make sure the data on the page you are trying to extract is actually loaded. Also, make sure to go to edit
and enable Add CORS Headers
to allow the admin's browser to make requests to the site.
window.addEventListener('load', () => {
// ... your code here
});